I don't know how you are using twitter, but I like to follow friends as well as news sites and politicians. Normally, you'll see your friends' timeline sorted by date. This can be annoying if you got some spammy friends that dominate your first page and make it hard to keep up with others that don't tweet that often.
I didn't find a client that is capable of reordering the timeline - taking into account how many tweets a friend is writing - so I decided to write it on my own.
The result is a Ruby on Rails application, which you can run on your computer (i don't plan on hosting it somewhere at the moment). It looks like this if you use Mozilla Prism to convert the website to a local application:
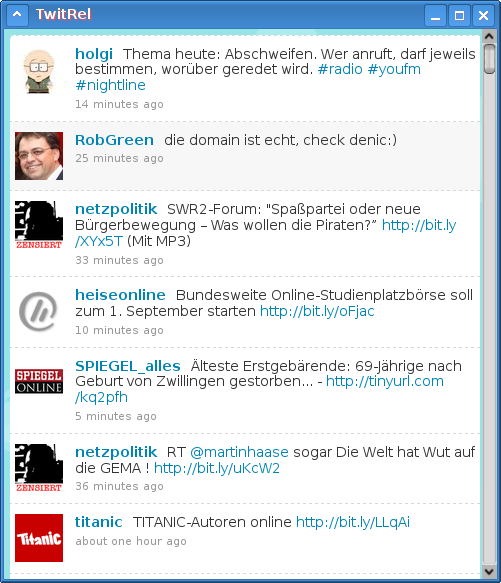
The code is available for downloading, forking or anything over at github.
The README file in the Repository can help you get up and running.
If you are interested in how the reordering works, I'll explain it shortly. The following code is taken from app/controllers/timeline_controller.rb:
We're connecting to twitter with the great Twitter4R API and then fetch 300 tweets from your friends' timeline. Next, the number of tweets per friend is calculated (in the current timeline).
Each tweet now gets a relevance score calculated by n0.6 × Δt, with n the number of tweets for the specific user ("tweep" that send the update) and Δt, the time interval passed since the status update.
0.6 is an arbitrary constant, you can test what best matches your taste (try some numbers between 0 and 1 to see the difference).
I didn't find a client that is capable of reordering the timeline - taking into account how many tweets a friend is writing - so I decided to write it on my own.
The result is a Ruby on Rails application, which you can run on your computer (i don't plan on hosting it somewhere at the moment). It looks like this if you use Mozilla Prism to convert the website to a local application:
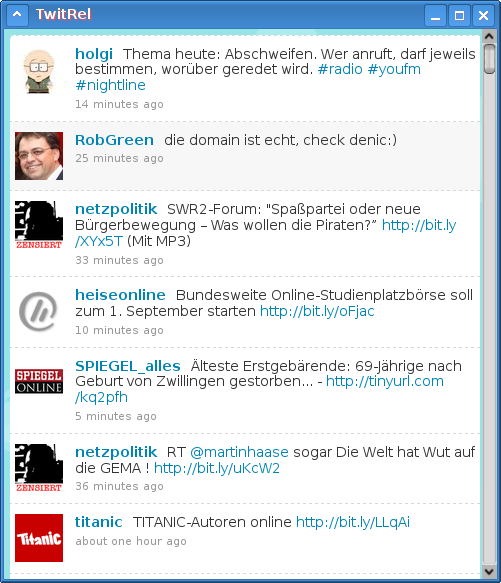
The code is available for downloading, forking or anything over at github.
The README file in the Repository can help you get up and running.
If you are interested in how the reordering works, I'll explain it shortly. The following code is taken from app/controllers/timeline_controller.rb:
twitter = Twitter::Client.new(:login => USER, :password => PASSWORD)
timeline = twitter.timeline_for(:friends, :count => 300)
my_id = twitter.my(:info).id
timeline = timeline.select { |entry| entry.user.id != my_id }
numtweets = {}
twitter.my(:friends).each do |friend|
num = timeline.select { |entry| entry.user.id == friend.id }.size
numtweets.store(friend.id, num)
end
@tweets = timeline.sort_by { |entry| (numtweets[entry.user.id] ** 0.6) * (Time.now - entry.created_at) }
timeline = twitter.timeline_for(:friends, :count => 300)
my_id = twitter.my(:info).id
timeline = timeline.select { |entry| entry.user.id != my_id }
numtweets = {}
twitter.my(:friends).each do |friend|
num = timeline.select { |entry| entry.user.id == friend.id }.size
numtweets.store(friend.id, num)
end
@tweets = timeline.sort_by { |entry| (numtweets[entry.user.id] ** 0.6) * (Time.now - entry.created_at) }
We're connecting to twitter with the great Twitter4R API and then fetch 300 tweets from your friends' timeline. Next, the number of tweets per friend is calculated (in the current timeline).
Each tweet now gets a relevance score calculated by n0.6 × Δt, with n the number of tweets for the specific user ("tweep" that send the update) and Δt, the time interval passed since the status update.
0.6 is an arbitrary constant, you can test what best matches your taste (try some numbers between 0 and 1 to see the difference).